The Evolution of Multiple Inheritance in Java
- Ruhi Parveen
- Dec 16, 2024
- 3 min read
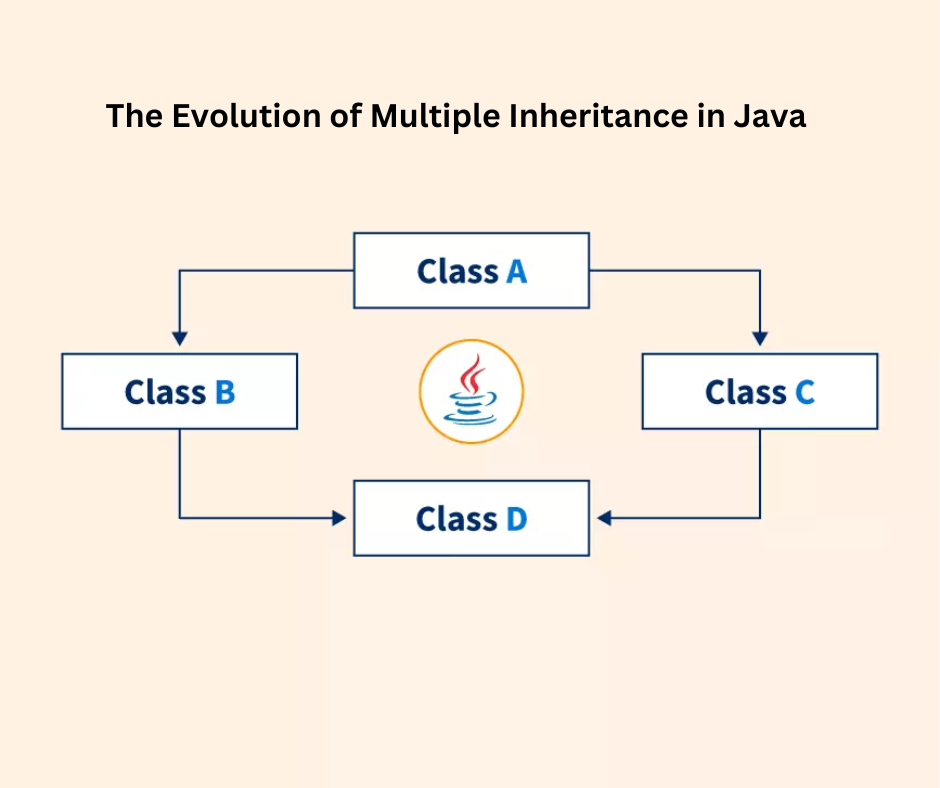
Multiple inheritance is a programming concept that allows a class to inherit attributes and behaviors from more than one parent class. While this concept has existed in other programming languages for decades, Java has taken a unique approach to managing and evolving multiple inheritance to avoid potential pitfalls. Here's an in-depth look at the evolution of multiple inheritance in Java.
What is Multiple Inheritance?
Multiple inheritance allows a class to inherit properties and methods from two or more classes. This feature can be beneficial in certain situations but comes with challenges, such as:
Ambiguity: When two parent classes have methods with the same signature, it can create conflicts.
Complexity: Maintaining a hierarchy with multiple parent classes can become complicated.
Java avoids these issues by not supporting multiple inheritance directly through classes but providing alternative solutions.
Java’s Early Approach to Inheritance
Java was designed as a simple, object-oriented, and robust language when it was introduced in the mid-1990s. To avoid the complications seen in languages like C++, Java did not support multiple inheritance through classes. Instead, it implemented single inheritance and provided the concept of interfaces to simulate multiple inheritance.
Interfaces: Java’s Initial Solution
In Java 1.0, interfaces were introduced as a solution to provide a form of multiple inheritance. Unlike classes, interfaces allow a class to implement multiple behaviors without creating ambiguity.
Key Features of Interfaces:
No State: Interfaces could only declare methods, and classes implementing those interfaces had to define the method bodies.
Multiple Implementations: A class could implement multiple interfaces, thus achieving a form of multiple inheritance.
This approach ensured simplicity and reduced the risks of ambiguity, making Java easier to learn and use compared to languages with full-fledged multiple inheritance.
The Limitations of Early Interfaces
Although interfaces solved some problems, they came with limitations:
Code Duplication: Developers often had to repeat the same code in multiple classes implementing the same interface.
Lack of Default Methods: Interfaces could not have any method implementation, requiring all methods to be implemented in the derived classes.
These limitations led to the evolution of Java’s approach to multiple inheritance over time.
Introduction of Default Methods (Java 8)
The release of Java 8 in 2014 marked a significant milestone in the evolution of multiple inheritance. To address the limitations of interfaces, default methods were introduced. These are methods with a predefined implementation inside interfaces.
How Default Methods Work:
A class that implements an interface can either use the default method implementation or provide its own override.
Default methods made it possible to add new functionality to existing interfaces without breaking the code of implementing classes.
This addition allowed Java to move closer to true multiple inheritance while maintaining its core philosophy of simplicity and clarity.
Conflict Resolution in Default Methods
With the addition of default methods, Java introduced a mechanism to handle potential conflicts:
Most Specific Rule: If a class inherits a default method from multiple interfaces, the method from the most specific interface is used.
Explicit Override: If ambiguity persists, the developer must override the method in the implementing class.
Static Methods in Interfaces (Java 8)
Java 8 also introduced static methods in interfaces. These methods could not be overridden by implementing classes, ensuring there was no confusion or conflict. This feature further strengthened the capabilities of interfaces in Java.
Emergence of Functional Interfaces and Lambdas
In Java 8, the concept of functional interfaces (interfaces with a single abstract method) gained prominence. These were integral to enabling lambda expressions, which simplified the way developers write and use functional-style code. This evolution highlighted the increasing importance of interfaces in modern Java development.
Multiple Inheritance Using Abstract Classes
While Java primarily relies on interfaces, abstract classes have also been a part of its inheritance model. Abstract classes can serve as a base class for other classes and allow shared code and state. However, a class in Java can only extend one abstract class, limiting their use for multiple inheritance scenarios.
Future Directions in Java
The evolution of multiple inheritance in Java reflects the language's adaptability to modern software development needs. Future updates may further refine inheritance models while prioritizing simplicity, readability, and performance.
Conclusion
The journey of multiple inheritance in Java has been one of thoughtful evolution. From its initial reliance on interfaces to the introduction of default and static methods in Java 8, the language has consistently found innovative ways to address the challenges of multiple inheritance without sacrificing simplicity. By balancing flexibility and clarity, Java continues to empower developers with tools that are powerful yet easy to use.
Comments